[LeetCode] 308. Range Sum Query 2D - Mutable
Given a 2D matrix matrix, find the sum of the elements inside the rectangle defined by its upper left corner (row1, col1) and lower right corner (row2, col2).
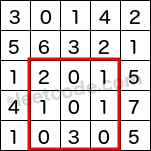
The above rectangle (with the red border) is defined by (row1, col1) = (2, 1) and (row2, col2) = (4, 3), which contains sum = 8.
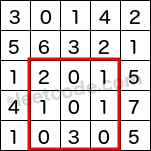
The above rectangle (with the red border) is defined by (row1, col1) = (2, 1) and (row2, col2) = (4, 3), which contains sum = 8.
Example:
Given matrix = [ [3, 0, 1, 4, 2], [5, 6, 3, 2, 1], [1, 2, 0, 1, 5], [4, 1, 0, 1, 7], [1, 0, 3, 0, 5] ] sumRegion(2, 1, 4, 3) -> 8 update(3, 2, 2) sumRegion(2, 1, 4, 3) -> 10
Note:
- The matrix is only modifiable by the update function.
- You may assume the number of calls to update and sumRegion function is distributed evenly.
- You may assume that row1 ≤ row2 and col1 ≤ col2.
Thought process:
Binary indexed tree.
Solution:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 | class NumMatrix { private int[][] matrix; private int[][] tree; private int height; private int width; public NumMatrix(int[][] matrix) { height = matrix.length; if (height == 0) { return; } width = matrix[0].length; this.matrix = new int[height][width]; tree = new int[height + 1][width + 1]; for (int i = 0; i < height; i++) { for (int j = 0; j < width; j++) { update(i, j, matrix[i][j]); } } } public void update(int row, int col, int val) { int diff = val - matrix[row][col]; matrix[row][col] = val; for (int i = row + 1; i <= height; i += (i & -i)) { for (int j = col + 1; j <= width; j += (j & -j)) { tree[i][j] += diff; } } } public int sumRegion(int row1, int col1, int row2, int col2) { return sum(row2, col2) - sum(row1 - 1, col2) - sum(row2, col1 - 1) + sum(row1 - 1, col1 - 1); } private int sum(int row, int col) { int sum = 0; for (int i = row + 1; i > 0; i -= (i & -i)) { for (int j = col + 1; j > 0; j -= (j & -j)) { sum += tree[i][j]; } } return sum; } } /** * Your NumMatrix object will be instantiated and called as such: * NumMatrix obj = new NumMatrix(matrix); * obj.update(row,col,val); * int param_2 = obj.sumRegion(row1,col1,row2,col2); */ |
Time complexity:
Say height = h and width = w.
- Constructor: hw logh logw.
- update(): logh logw.
- sumRegion(): logh logw.
Comments
Post a Comment